In our previous articles, we have implemented Basic Authentication, JWT Authentication, LDAP authentication with BASIC_AUTH
, JWT_AUTH
and LDAP_AUTH
profiles respectively.
When we run the the application with any of the above profile, then the respective implementation of WebSecurityConfigurerAdapter
class will get registered.
If we don’t specify any of the above profile, then the browser will redirect to the default login page and prompt for credentials as shown below
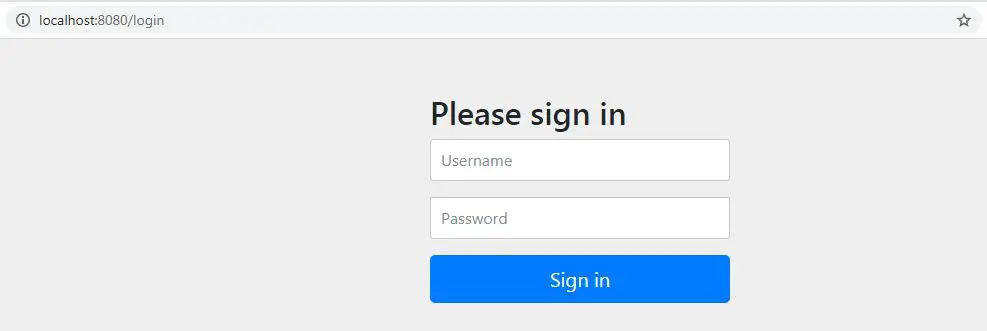
In case if we don’t need authentication for a Junit test suite, then we should be able to disable Spring Security for those use cases. Hence, we are gonna add a NO_AUTH
Profile and disable Spring Security for that profile alone.
Configure Spring Security with No Authentication
Profiles.java
Create a constant for No Authentication profile
package com.javachinna.config;
public class Profiles {
private Profiles() {
}
public static final String NO_AUTH = "noauth";
public static final String BASIC_AUTH = "basicauth";
public static final String JWT_AUTH = "jwtauth";
public static final String LDAP_AUTH = "ldapauth";
}
NoAuthSecurityConfig.java
@Profile(Profiles.NO_AUTH)
annotation is used to disable authentication only when the application is run with “noauth
” profile.
NoAuthSecurityConfig
class extends the WebSecurityConfigurerAdapter
which is a convenience class that allows customization to both WebSecurity and HttpSecurity.
package com.javachinna.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
@Profile(Profiles.NO_AUTH)
public class NoAuthSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity httpSecurity) throws Exception {
// Disable CSRF
httpSecurity.csrf().disable()
// Permit all requests without authentication
.authorizeRequests().anyRequest().permitAll();
}
}
Run with No Auth Profile
You can run the application using mvn spring-boot:run -Dspring-boot.run.profiles=noauth
and hit the url http://localhost:8080/swagger-ui.html in the browser. You should be able to execute the services without authentication.
Disable Security for JUnit Tests
You can run JUnit tests without authentication by specifying NO_AUTH
profile in the @ActiveProfiles
annotation as shown below
@SpringBootTest
@ActiveProfiles(Profiles.NO_AUTH)
class SpringBootRestCrudApplicationTests {
}
Source Code
As always, you can get the source code from the Github below
https://github.com/JavaChinna/spring-boot-disable-security
Conclusion
That’s all folks! In this article, you’ve learned how to disable authentication for Spring Boot RESTful services.
I hope you enjoyed this article. Thank you for reading.